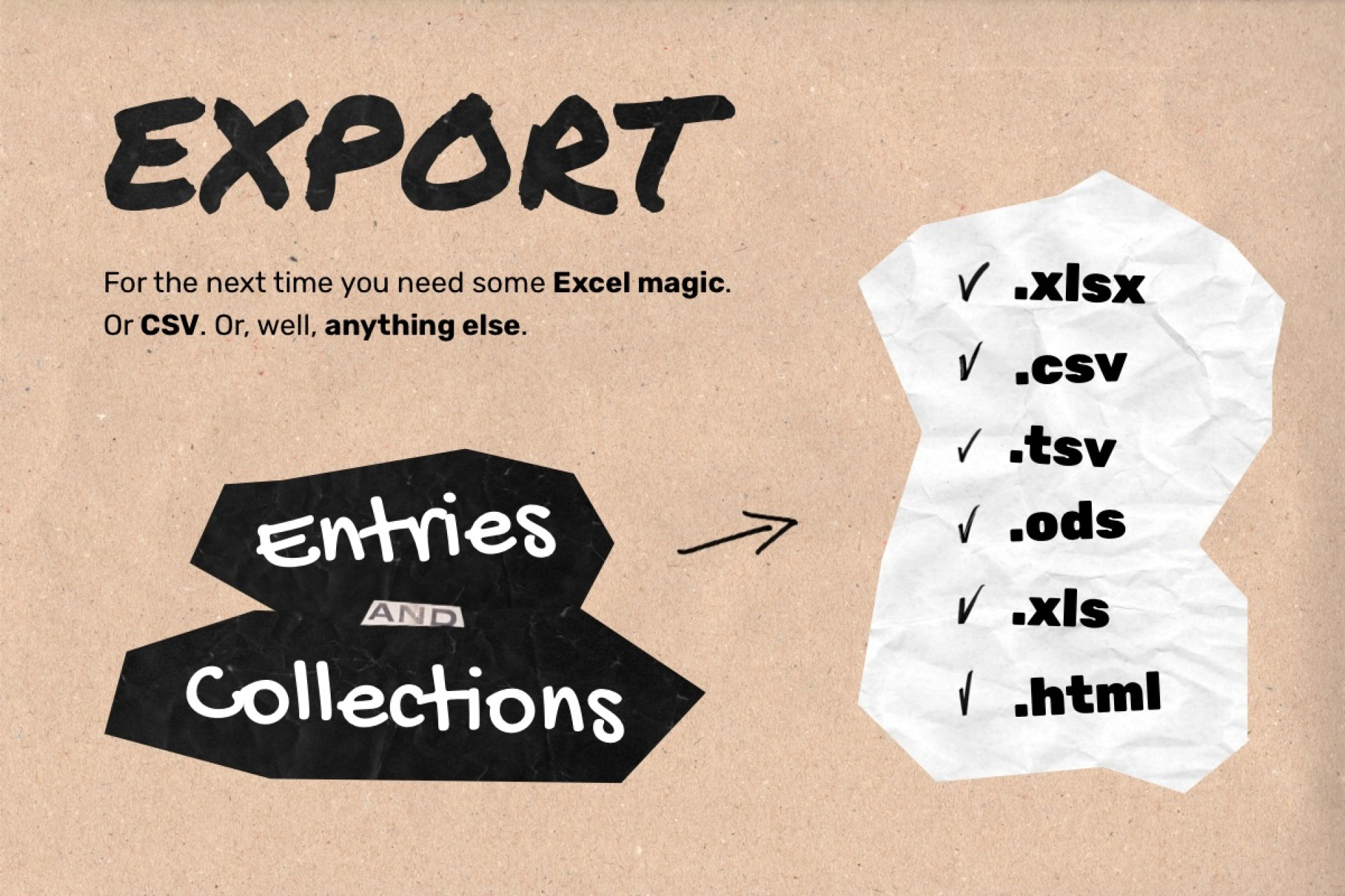
"Export" - A Statamic addon to export collections and entries to any format
Export is a neat Statamic addon that does pretty much exactly what you would expect. It exports stuff. More specifically you may choose to export certain entries when browsing a collection or you may also export entire collections using the provided utility.
Why would you need this?
Let's see two examples where this addon might come in handy.
Website Migration or Backup: Suppose a web developer or content manager is tasked with migrating content from one Statamic site to another platform or simply needs to create a comprehensive backup of all the site's content. Using this addon, they could export entire collections or selected entries in CSV or XLSX format. This would allow for an easy transfer to another CMS that supports data import from these formats, or for keeping a structured, offline copy of the site's content for archival purposes. Which is my personal favorite.
Event Management and Reporting: Imagine an organization that uses Statamic to manage events, including registrations, attendee information, and session details. Prior to an event, the team needs to organize session assignments, prepare name tags, and ensure catering counts are accurate. Using Export, they can export the relevant collections (e.g., registrations, sessions) to XLSX or CSV format. This data can then be used to:
Create personalized agendas for attendees by matching session registrations with session details.
Generate name tags by extracting attendee names and affiliations.
Share precise attendee numbers with caterers and venue managers to manage logistics.
Alright, so how to use this addon. There are two main ways to get your data in the format you need.
Exporting individual entries
To export entries from a given collection all you need to do is check the boxes of the entries you'd like to export, adjust the settings (or keep the defaults) and click "Export entries".
Export entire collections
While exporting individual entries is straight forward, exporting collections is just as easy. Head over to the "Export" section of the utilities, select the corresponding settings if you want to and done.
How it's done
Under the hood I'm using a very neat package called Laravel Excel. If you ever need to create or modify an Excel sheet you should definitely check this one out. It support other file types like CSV as well.
So there are several ways on how you can create an Excel file using Laravel Excel. Let's look at a piece of my code I wrote when building this addon.
1<?php 2 3namespace Doefom\StatamicExport\Exports; 4 5... 6 7class EntriesExport implements FromCollection, WithStyles 8{ 9 ...10 11 /**12 * Get all keys from all items combined (unique). Then go through all keys and check for each item if the key13 * exists and if it does store the value. Else, store an empty string.14 *15 * If headers should be included, prepend those to the result array.16 *17 * @return Collection18 */19 public function collection(): Collection20 {21 // Get all unique keys from all items22 $keys = $this->getAllKeysCombined($this->items);23 24 $result = [];25 foreach ($keys as $key => $label) {26 // Add the key to the collection if it doesn't exist27 foreach ($this->items as $index => $item) {28 // If the key doesn't exist, add an empty string to avoid unnecessary augmentation.29 if ($item->get($key) === null) {30 $result[$index][$key] = ''; // Necessary to prevent mixing up columns31 continue;32 }33 $value = $item->augmentedValue($key);34 $result[$index][$key] = $this->toString($value);35 }36 }37 38 // Add the headers to the collection39 if (Arr::get($this->config, 'headers', true)) {40 $result = array_prepend($result, $keys->toArray());41 }42 43 return collect($result);44 }45 46 ...47 48}
When using Laravel Excel you can define export classes wich must have a collection method that returns a collection. The cool thing is, that's all you need to do. Return the collection you want to have in your export and you're good to go. So let's walk through the above code.
Retrieving all keys of the entries present in the export
1// Get all unique keys from all items2$keys = $this->getAllKeysCombined($this->items);
First of all we need to know what fields (or columns) we will have in the export. I wrote a helper function to walk through all the entries the user selected, get all the keys and return an array of unique keys. We need to do this because entries within a collection can have different blueprints and therefore can have different fields. To support exporting any collection and any combination of entries within a collection, we need to collect all the keys present in any of the blueprints.
Looping through the keys and retrieving the values for each entry
1$result = []; 2foreach ($keys as $key => $label) { 3 // Add the key to the collection if it doesn't exist 4 foreach ($this->items as $index => $item) { 5 // If the key doesn't exist, add an empty string to avoid unnecessary augmentation. 6 if ($item->get($key) === null) { 7 $result[$index][$key] = ''; // Necessary to prevent mixing up columns 8 continue; 9 }10 $value = $item->augmentedValue($key);11 $result[$index][$key] = $this->toString($value);12 }13}
Here we loop through the keys we extracted earlier. For each key we also loop through every entry we want to export. We do it this way to ensure that every column is present for every row (entry). If we would do it the other way round, some rows might not have all the columns (if more than one blueprint is present) which would result in a messy and mixed up export. Note the line $result[$index][$key] = '';
which is used to set a default value of an empty string if the key is not found or the value is null. An empty string results in an empty cell and that's what we want in this case.
1// Add the headers to the collection2if (Arr::get($this->config, 'headers', true)) {3 $result = array_prepend($result, $keys->toArray());4}5 6return collect($result);
Lastly we check if the user checked the configuration option to add the header columns to the export which are just the column names. That's the default setting but this can be disabled as well. And yeah, that's pretty much it. If you want to check out the exact code and for example how the getAllKeysCombined
function looks like feel free to take a look at the repo on GitHub. You find it here: https://github.com/doefom/statamic-export
Conclusion
During my career as a web developer I came across many occasions where having some kind of data available in Excel would have been great or would have made my life easier. Or actually it would have made mine and my client's life easier. The less "techy" an industry is, the more likely it is Excel is used throughout the company working in this industry. I guess these are especially the cases where this addon might help you get to your results faster.
Thanks a lot for reading and see you soon!